3. Make your ModelVersion Deployable
On this page, we will show you how to deploy a ModelVersion
on the Picsellia Serving Engine. This is particularly relevant if the model has been imported to Picsellia through its Model files, as detailed on the previous page.
Please note that the guidance and policies to follow when deploying aModelVersion
depend on the Framework used by the ModelVersion
.
Can I deploy the
ModelVersion
on my own infrastructure, but leverage Picsellia monitoring?If you do not want to use Picsellia Serving Engine for your
ModelVersion
and just enable its monitoring, follow the documentation here.
1. Make a TensorFlow ModelVersion
Deployable
TensorFlow ModelVersion
Deployable- Train your model with
Tensorflow
. - Export your model to a folder (here is the Tensorflow documentation on model export). Your folder should resemble the one shown below:
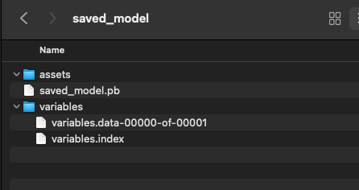
- Zip this folder (here, the folder is named saved_model). Note that the model folder must be zipped before being uploaded it to Picsellia.
- Upload the Model files in the
ModelVersion
with the script below:
from picsellia import Client
from picsellia.types.enums import InferenceType
client = Client(api_token=api_token, organization_name=organization_name)
model_name = "efficientdet_d0_coco17_tpu-32"
my_model = client.get_model(
name=model_name,
)
my_model_version = my_model.get_version(
name='v1',
)
my_model_version.store("model-latest", "path/to/saved_model.zip")
That's it! Your TensorFlow ModelVersion
is now ready to be deployed, you should see a Deploy button appearing on your _ModelVersion overview _page:
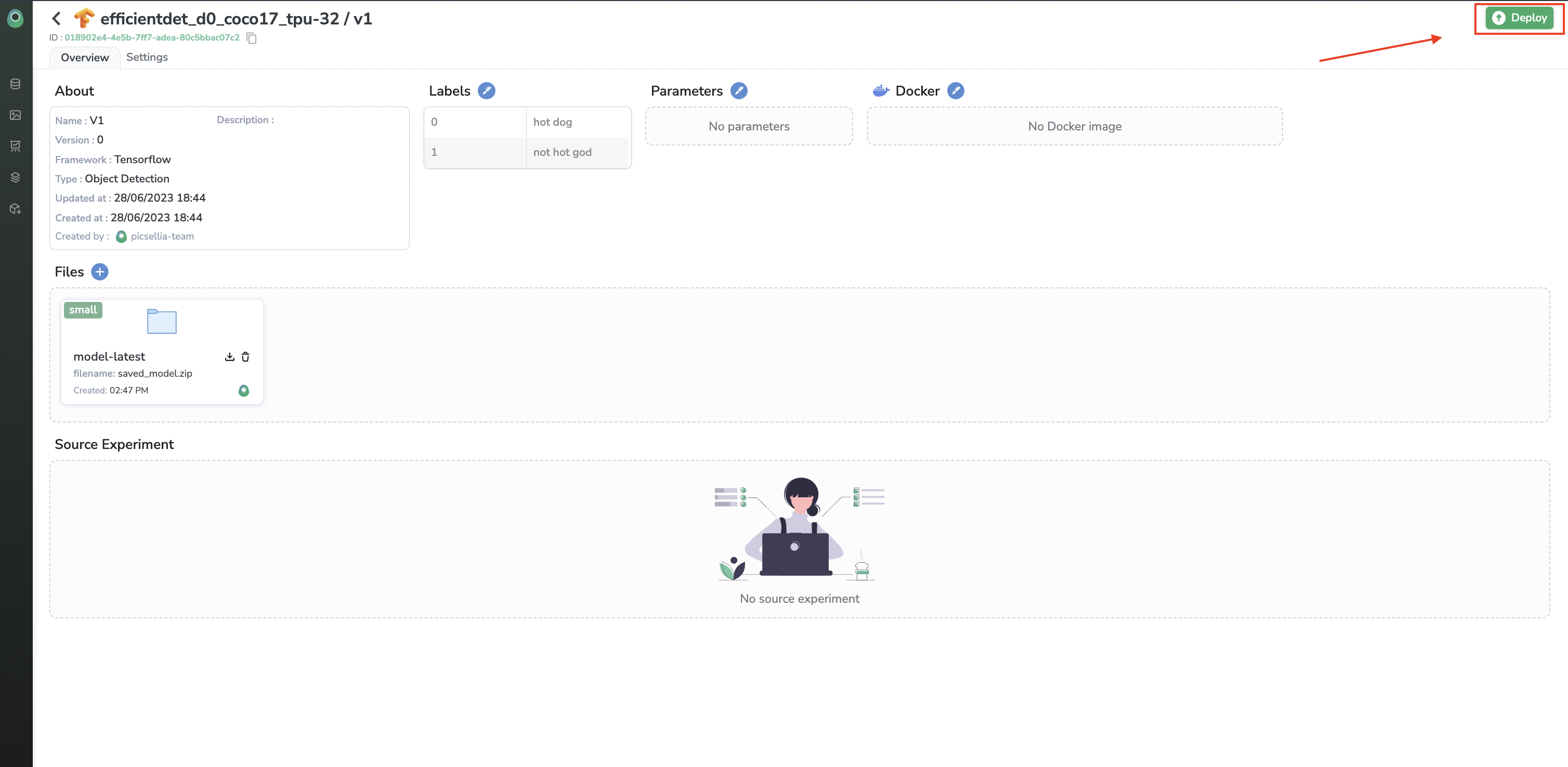
2. Make a PyTorch ModelVersion
Deployable
PyTorch ModelVersion
DeployableDeploying a PyTorch
model with Picsellia requires an ONNX ModelVersion
.
- (Optional) If you don't have one, create an ONNX
ModelVersion
:
from picsellia import Client
from picsellia.types.enums import InferenceType
client = Client(api_token=api_token, organization_name=organization_name)
model_name = "pytorch-model"
my_model = client.create_model(
name=model_name,
)
## Define the model version Inference type
inference_type = InferenceType.OBJECT_DETECTION
## Define the model version LabelMap
labelmap = {
'0': 'avocado',
'1': 'not avocado'
}
##Define the model version Framework
framework = Framework.ONNX
my_model_version = my_model.create_version(
name='v1',
labels=labelmap,
type=inference_type,
framework=framework
)
- Train your model with
PyTorch
. - Export your model
PyTorch
model into an ONNX model to a folder (here is the PyTorch documentation on ONNX model export). - Upload the Model files in the
ModelVersion
with the script below:
from picsellia import Client
from picsellia.types.enums import InferenceType
client = Client(api_token=api_token, organization_name=organization_name)
model_name = "pytorch-model"
my_model = client.get_model(
name=model_name,
)
my_model_version = my_model.get_version(
name='v1',
)
my_model_version.store("model-latest", "path/to/model.onnx")
That's it! Your PyTorch ModelVersion
is now ready to be deployed through ONNX, you should see a Deploy button appearing on your ModelVersion
overview page:
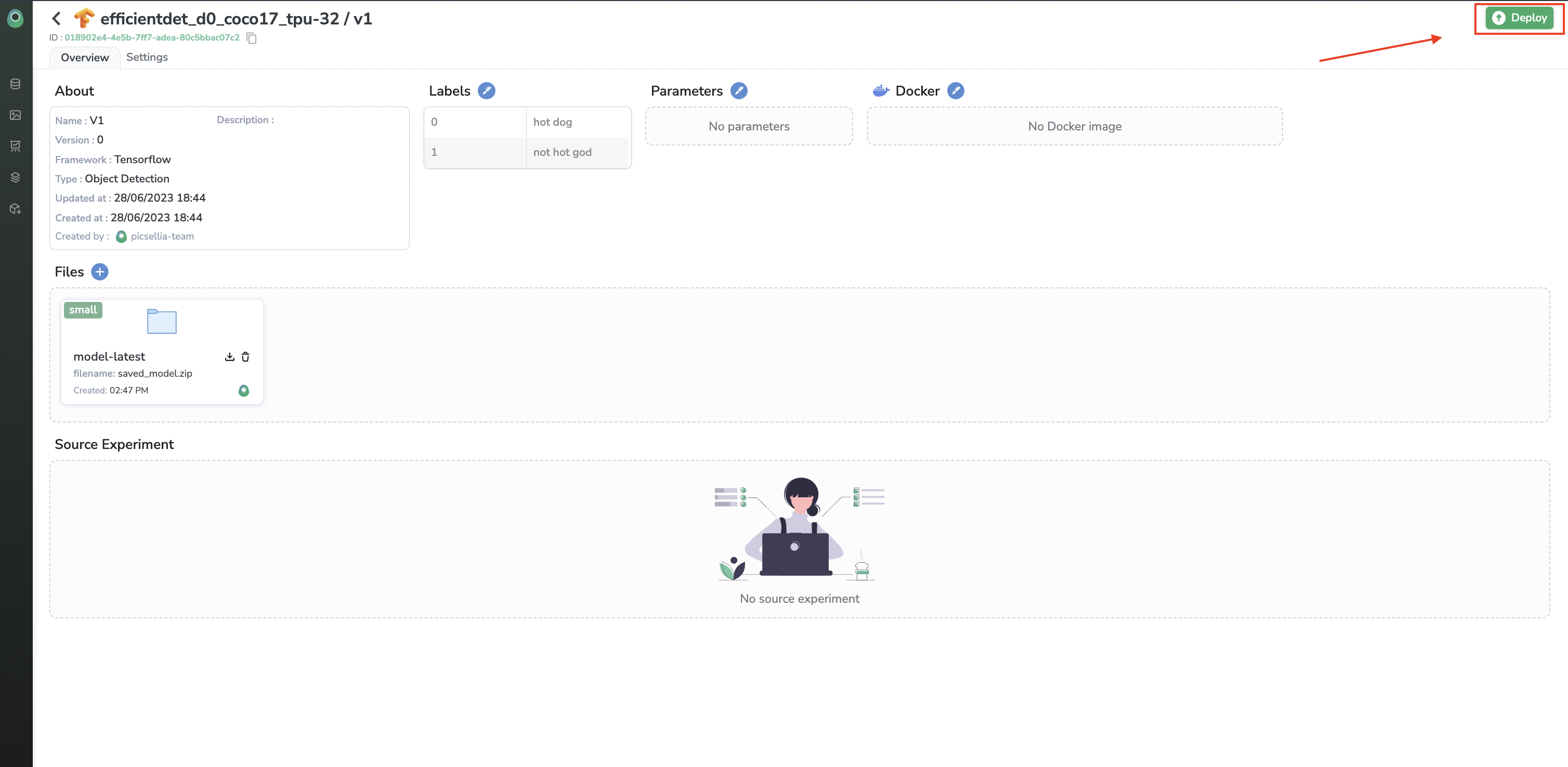
3. Make an ONNX ModelVersion
Deployable
ModelVersion
Deployable- Train your model with
ONNX
. - Export your model to a folder.
- Upload the Model files in the
ModelVersion
with the script below:
from picsellia import Client
from picsellia.types.enums import InferenceType
client = Client(api_token=api_token, organization_name=organization_name)
model_name = "efficientdet_d0_coco17_tpu-32"
my_model = client.get_model(
name=model_name,
)
my_model_version = my_model.get_version(
name='v1',
)
my_model_version.store("model-latest", "path/to/model.onnx")
That's it! Your ONNX ModelVersion
is now ready to be deployed, you should see a Deploy button appearing on your ModelVersion overview page:
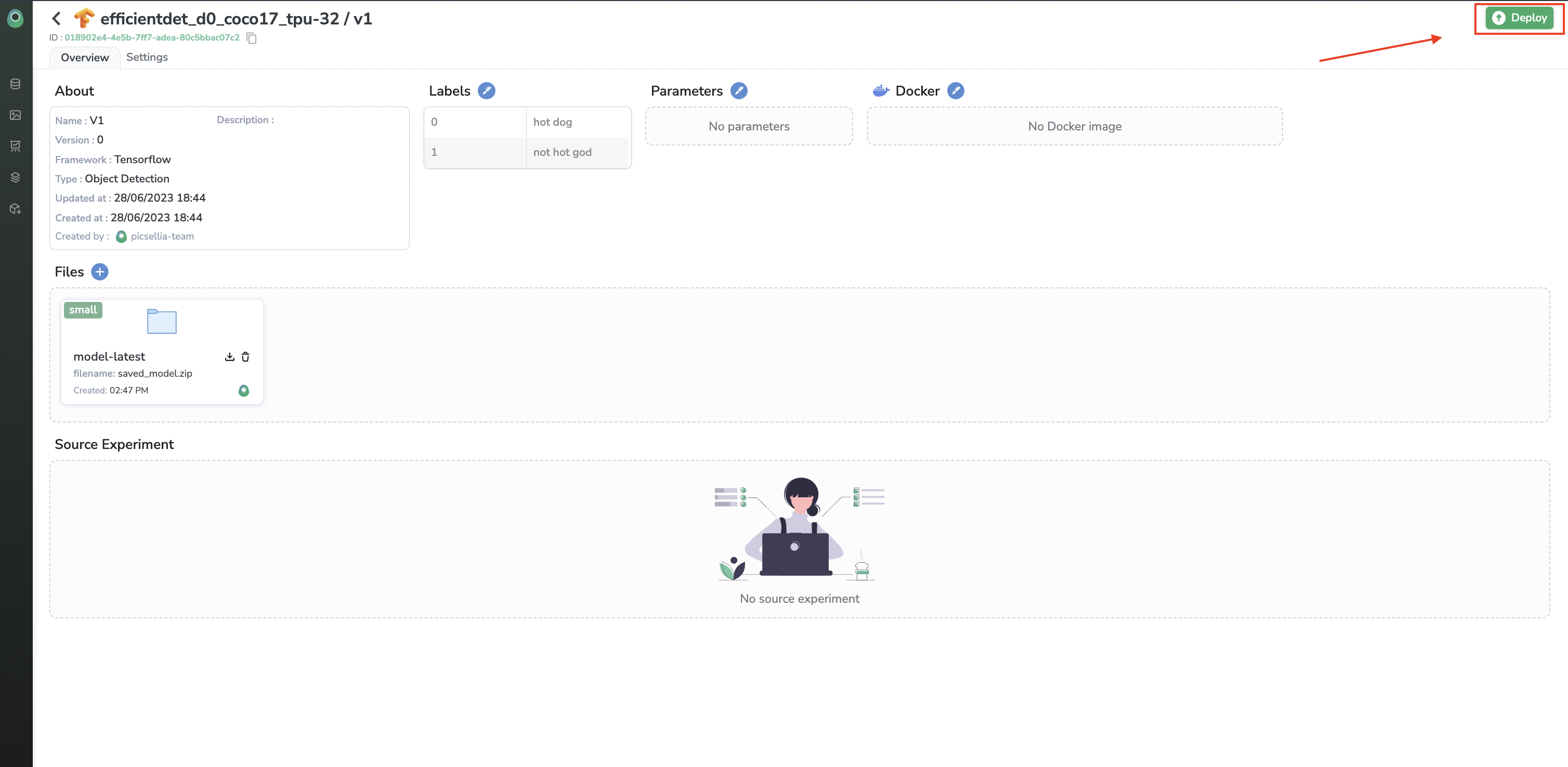
4. Monitoring
Your model is now fully uploaded to Picsellia as a ModelVersion
. It has been deployed on the Picsellia Serving Engine and is ready to make inferences, as described in this recipe:
Once predictions have been made, you can use the Picsellia Monitoring Dashboard to monitor the ModelVersion
's performance.
If the ModelVersion
performance starts to decay due to data drift, the best way to anticipate and fix this common issue is to retrain the ModelVersion with a DatasetVersion
enriched with Prediction
from the production ground, reviewed by a human. The implementation of this process is well detailed in our Quick Start Guide.
Updated 14 days ago