4. Track your trainings with Callbacks
Now that we have retrieved all necessary inputs from Picsellia, we are almost ready to launch the training of our Experiment
. However, before launching the training, we need to define the metrics we want to track.
During the training process, you can gather metrics using callbacks. To ensure that these metrics are saved in the Picsellia Experiment
instead of locally, we need to customize the callbacks to adapt them to Picsellia.
For example, we can create a custom Keras Callback to log the value generated for every epoch. To do this, we need to adapt the callback to use LogType
from the Picsellia package.
For guidance on creating Keras callbacks, see the Keras documentation on callbacks.
from picsellia import Experiment
from picsellia.types.enums import LogType
import keras
class CustomPicselliaCallback(keras.callbacks.Callback):
def __init__(self, experiment: Experiment) -> None:
self.experiment = experiment
def on_epoch_end(self, epoch, logs=None):
for k, v in logs.items():
try:
self.experiment.log(k, list(map(float, v)), LogType.LINE)
except Exception as e:
print(f"can't send {v}")
Here we just instantiate a CustomCallback in charge of Logging your results.
Now it's time to add it to your callback list and let the magic happen.
# ...
class CustomPicselliaCallback(keras.callbacks.Callback):
def __init__(self, experiment: Experiment) -> None:
self.experiment = experiment
def on_epoch_end(self, epoch, logs=None):
for k, v in logs.items():
try:
self.experiment.log(k, list(map(float, v)), LogType.LINE)
except Exception as e:
print(f"can't send {v}")
picsellia_callback = CustomPicselliaCallback(experiment=experiment)
epochs = parameters['epochs']
hist = model.fit(ds_train, epochs=epochs, validation_data=ds_test, verbose=2, callbacks=[picsellia_callback])
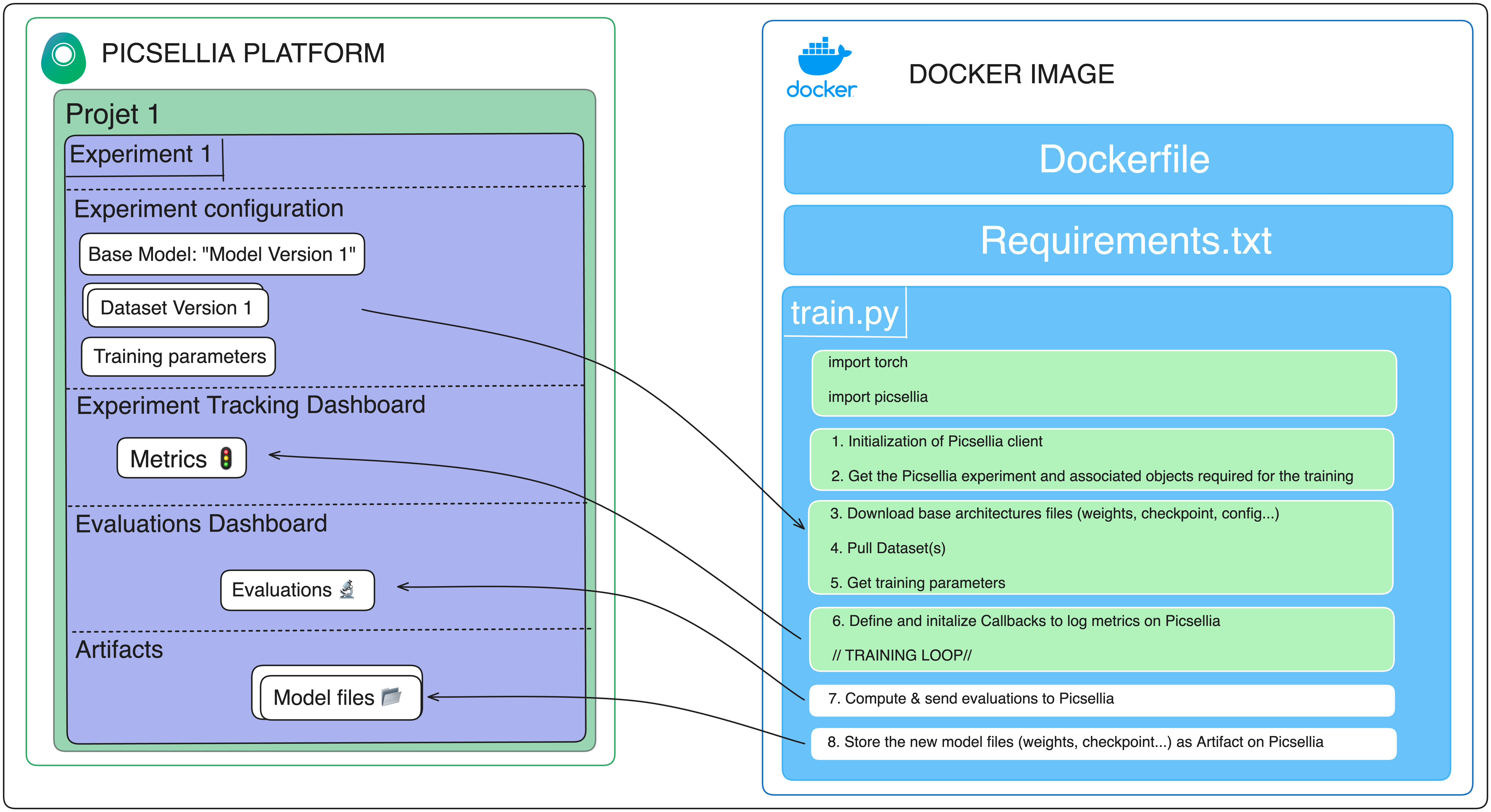
You can define as many callbacks as you want and log them in your Picsellia Experiment
as a LogType
.
Once this is done, you can launch the training loop on the training infrastructure.
Updated over 1 year ago